Programming Widget Layout
Use the gained knowledge to create forms.
Experimenting with QHBOXLayout
Create a project called hbox
with the following code:
int main(int argc, char* argv[])
{
QApplication app(argc, argv);
QWidget* window = new QWidget();
window->setWindowTitle("QHBoxLayout Test");
window->show();
return app.exec();
}
This will show an empty window. Your goal is to modify the code in order to display the following form:
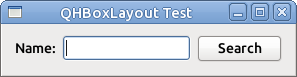
Nested Layouts
The goal of the exercice is learn to analyse the construction of a form and thencode it using Netsted layouts.
Here we show you a classic dialog from the book GB to search for a user.
You do not have to code any functinality, just the form of the dialog.
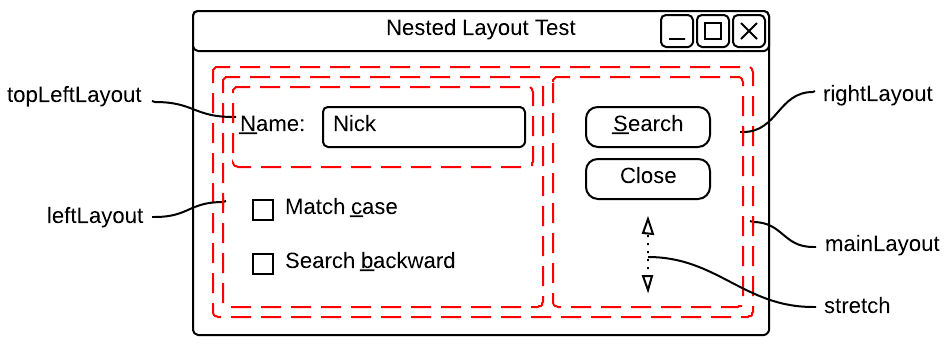
- In order to add a layout to a main one, you’ll have to use
addLayout(layout)
- The vertical space (Stretch) could be added by
layout->addStretch(dimension)
layout->addSpacer(SpaceItem)
- the Widget with a empty checkable square is a QCheckBox
Bug report Form
This example is taken from Qt Tutorial. You task is to create the following form to report a problem.
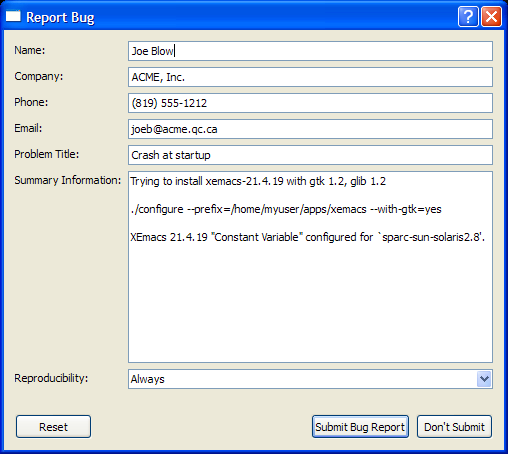
Grid Layout
For our final exercice, we will visit an imporant layout that we missed in class.
-
Check and read the documentation for the QGridLayout
-
Once you’ve read it, try to construct the following calculator:
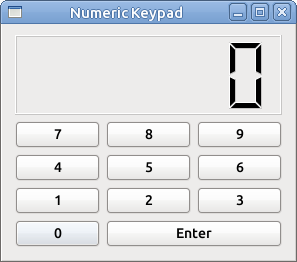
- The component showing the number is called a LCDNumber
- You may assume that at max it can contains 6 digits.
- Call the method
setMinimumHeight
on you LCDNumber to set a minimu height of 80 pixels.
Again we only want the form, in your next lab you will implement a functional calculator.