Colliding Mice
Draw a mouse using QPainter
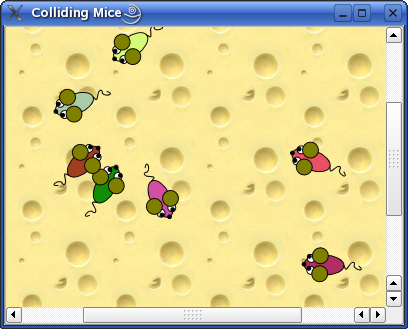
Overview
The goal of this homework is to use you gained knowledge using the QPainter
to
complete the colliding mice example. The solution has already the correct code. You will be an incpemplete version with an text
agent (simply showing the text). Your mission (if you choose to accept it) is the implement the
Mouse::paint(QPainter *painter, const QStyleOptionGraphicsItem *, QWidget *)
to draw the mouse.
Initial configuration
Download the initial project colliding.zip .
If you investigate the code of the Mouse
class, you’ll find that that painter
only draw a text instead of the form of the mouse.
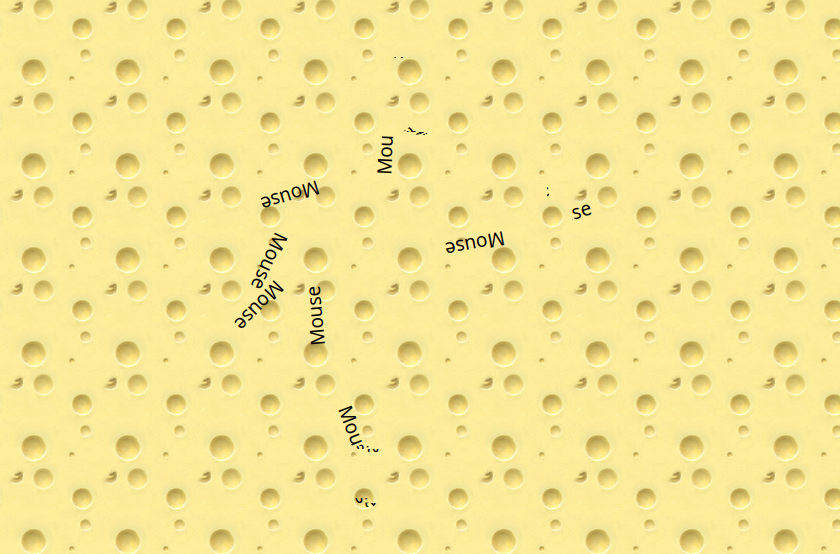
Drawing the mouse
Now you should fill the method
void Mouse::paint(QPainter *painter, const QStyleOptionGraphicsItem *, QWidget *)
{
// Body (Construct the boy of the mouse)
// Eyes
// Nose
// Pupils
// Ears
// Tail ( You'll need Bezier curve for that)
}
Transformations
The class QPainter
offers a set of basic transformation like:
scale(sx, sy)
to scale the catesian coordinates by sx and sy.rotate(angle)
to rotate axis byangle
.translate(dx, dy)
: to translate the axis by the vector (dx, dy)
To touch the power of those transformations try to produce the following image:
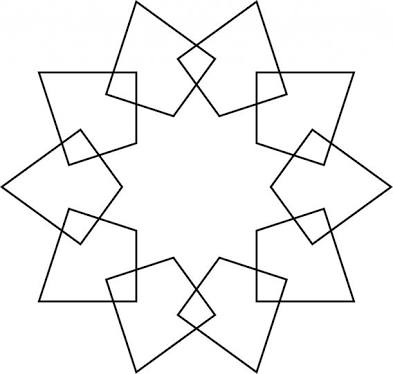
Try to apply a translation to have the center O in the cener of the widget
...
auto h = height();
auto w = width();
painter->translate(w/2, h/2);
//Draw your shapes now
For a second example, you should apply both rotation and scaling
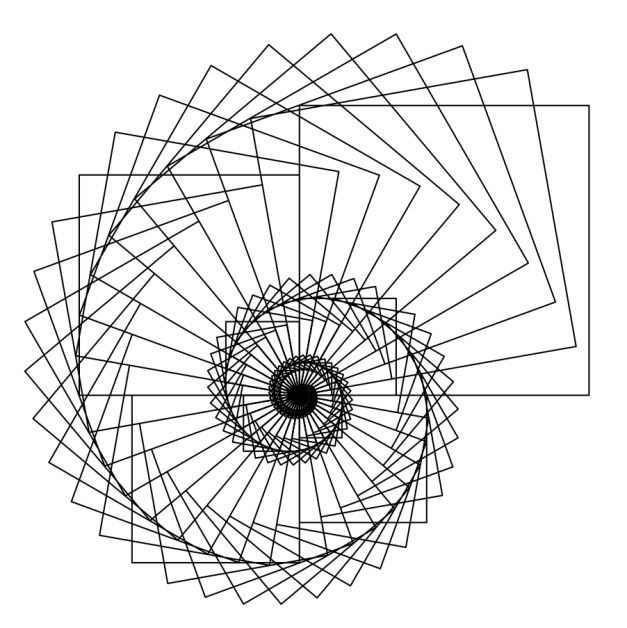
Fractral (revisit)
To check if you didn’t forget your knowledge about recurrence try to produce the following fractal.
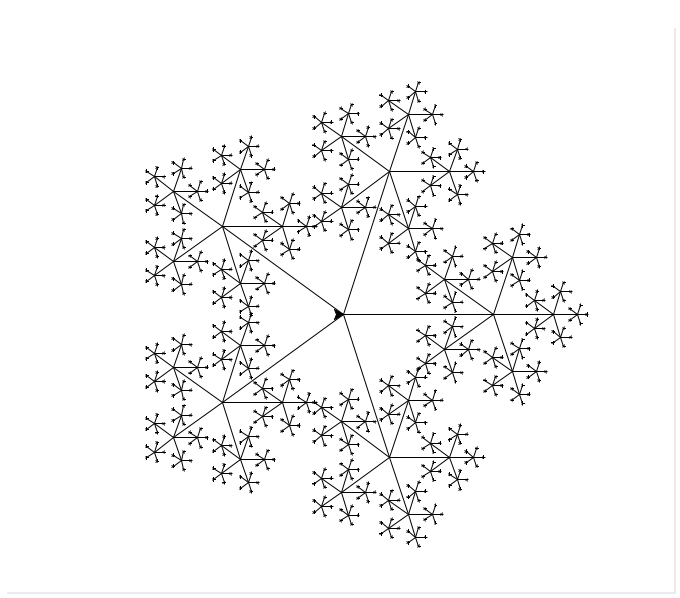
Here is an illustration to show the recursive pattern:
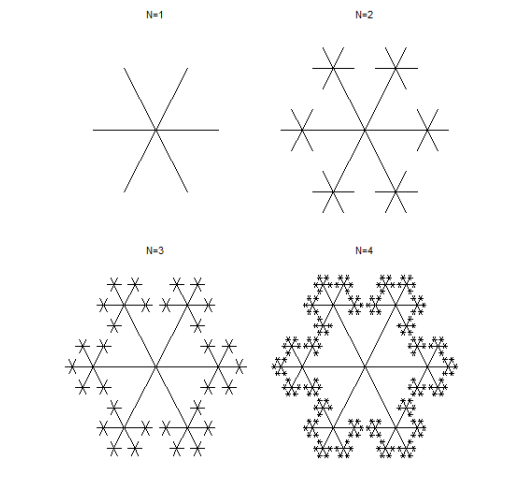